Code Block Cheat Sheet
This blog uses code blocks to display code samples. The Rehype Pretty Code plugin provides beautiful code blocks for your MD & MDX files. You can you choose from a number of preconfigured syntax highlighting themes or you can define your own one using a json file.
I use the Winter is Comming (Dark Black) custom theme from John Papa including the custom font DankMono on this page, which is my preferred theme in my local environment as well. The following list of code blocks shows different configurations by example.
JAVA Code Block
/HelloWorld.java
public class HelloWorld
{
public static void main (String[] args)
{
// Output "Hello World!"
System.out.println("Hello World!");
}
}
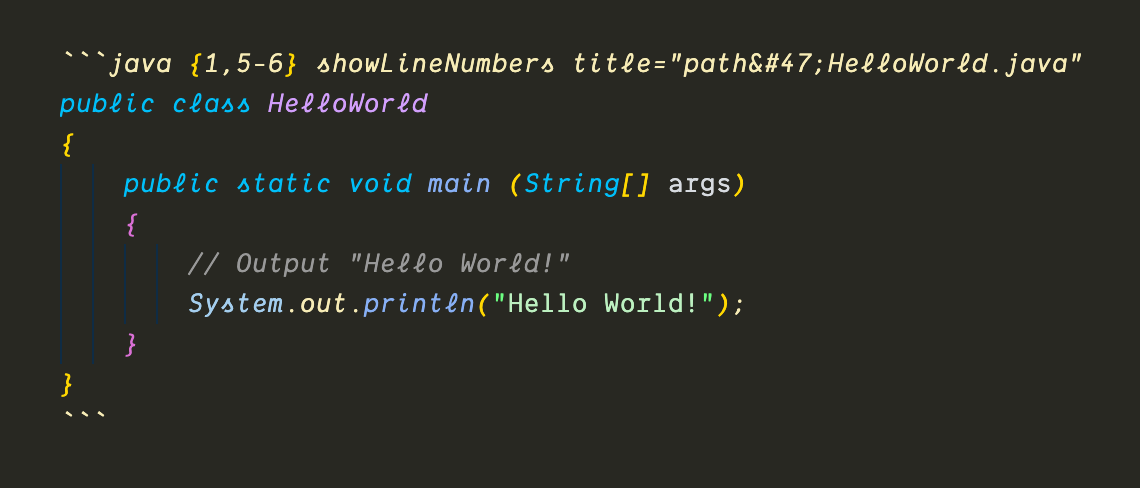
java
defines the code highlighter for the code block. The language will be shown as flag in the upper right corner as well.{1,5-6}
defines the rows that should be highlighted.showLineNumbers
defines that the line numbers will be shown on the left hand side.title="path/HelloWorld.java"
defines the name of the title in the top of the code block where/
is the sequence for the forward slash in html.
Terminal Block
Run this command ls -l
.
macOS:~$ ls -l
total 1408
drwxr-x---+ 84 root admin 2688 Nov 22 08:50 .
drwxr-xr-x 6 root admin 192 Nov 11 13:02 ..
-rw-r--r--@ 1 user staff 32772 Nov 21 20:48 .DS_Store
drwx------+ 9 user staff 288 Nov 11 14:11 Documents
-r-------- 1 user staff 7 Mar 7 2022 README.md
macOS:~$ _
Run this command to achive unattended happyness and control.
macOS:~$ rm -rf *
JavaScript Code Block
/fibonacciSequence.js
const number = parseInt(prompt('Enter the number of terms: '));
let n1 = 0, n2 = 1, nextTerm;
console.log('Fibonacci Series:');
for (let i = 1; i <= number; i++) {
console.log(n1);
nextTerm = n1 + n2;
n1 = n2;
n2 = nextTerm;
}
C Code Block
/fibonacciSequence.c
#include <stdio.h>
int main() {
int i, n;
// initialize first and second terms
int t1 = 0, t2 = 1;
// initialize the next term (3rd term)
int nextTerm = t1 + t2;
// get no. of terms from user
printf("Enter the number of terms: ");
scanf("%d", &n);
// print the first two terms t1 and t2
printf("Fibonacci Series: %d, %d, ", t1, t2);
// print 3rd to nth terms
for (i = 3; i <= n; ++i) {
printf("%d, ", nextTerm);
t1 = t2;
t2 = nextTerm;
nextTerm = t1 + t2;
}
return 0;
}